The Magazine
👩‍💻 Welcome to OPIT’s blog! You will find relevant news on the education and computer science industry.
Search inside The Magazine
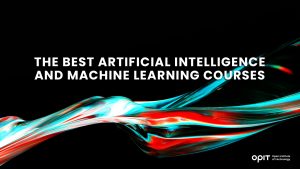
Every time you’ve chatted with a bot on a website, you’ve seen the basis of artificial intelligence (AI) and machine learning (ML) in action. Your experiences with augmented reality, any prompts you’ve ever delivered to ChatGPT, and a host of other technologies that businesses are already leveraging show us how crucial these two fields are, both today and in the future.
AI and ML are taking over the world. And with the right AI & ML courses, you put yourself in the ideal position to forge a career in an industry that’s set for a continuous annual growth rate of 36.2% between 2023 and 2030.
Factors to Consider When Choosing an AI and ML Course
AI ML courses come in all shapes and sizes, with some delivering the basics you need to build a foundation in the subjects and others moving on from those foundational concepts and into specializations. These five things are your biggest considerations when choosing a course.
1 – Course Content and Curriculum
What does the course teach? That’s not just an important question in terms of figuring out if the course helps you develop the skills you need, but it’s a crucial one for your future career prospects. The curriculum informs every step you take on your learning journey. If the content isn’t up to scratch (or takes you in a different direction than the one you intend to go in) it’s not the course for you.
2 – Course Duration and Flexibility
Combine work and family with your personal life and existing educational commitments and you have the recipe for a quagmire of time-consuming tasks that may not fit with a long-term course. The best AI and ML courses online offer flexibility, allowing you to fit your studies around other commitments and opening the door to self-paced learning.
3 – Your Instructors
Imagine you walk into a classroom and your instructor introduces themself. They tell you they have a couple of qualifications in the fields of AI and ML, but they haven’t worked in either industry and can’t expose you to professionals who have. Is that any use to you in your career? A good instructor combines technical expertise (which they’ll need a lot of) with industry experience they can draw on to lead you down the right career path.
4 – Course Reviews and Ratings
As any online marketer will tell you, user-generated content in the form of reviews, social media posts, and simple ratings tells you a ton about what a product delivers. That’s as true for AI and machine learning courses as it is for anything else. Check out what other people have to say about the course, paying special attention to former students and what’s happened to them in the wake of earning their certification.
5 – Pricing and Affordability
Money is always a challenge when it comes to education. Some universities charge tens of thousands of euros for their courses, which is fine if you can commit time and money to a full-time educational experience. It’s not so fine if you’re working on a budget. Your course’s cost plays a huge role in determining whether you take it. Just remember one thing – people tend to get what they pay for (for better or worse).
Top AI and ML Online Courses
Machine learning and artificial intelligence courses run the gamut from fast, industry-led courses designed to get you into a job to deeper degrees designed to equip you with everything you need to advance in your career. The following four are some of the best AI and ML courses online.
Course 1 – Master in Applied Data Science & AI (OPIT)
Designed for those at the postgraduate level, this Master’s degree requires you to have a background in computer science (or a relevant alternative). It’s a 100% online course that delivers an accredited degree under the European Qualification Framework (EQF), with the course also counting toward the college credits you may need to apply for future courses. Tutors are available for direct learning 24/7 and you learn via both recorded and live content delivered over the web.
Key Features and Benefits
- Offers tons of exposure to how machine learning and artificial intelligence apply in real-world scenarios
- You get a Master’s degree from a fully-accredited institution
- Favors progressive assessments over high-stress exams
- Control your own learning by arranging the course’s modules around your schedule
Enrollment Details
OPIT’s Master in Applied Data Science & AI comes in two flavors – the regular 18-month variety and a fast-tracked 12-month course. Enrollment is annual, with intake occurring every October, and the price varies depending on when you apply. Early birds get an extensive discount, paying €4,950 compared to the regular price of €6,500. You’ll need a relevant Bachelor’s degree in a subject like computer science to apply.
Course 2 – Machine Learning Introduction for Everyone (IBM via Coursera)
If OPIT’s Master’s degree is for people who are already halfway through the metaphorical marathon of machine learning and AI, IBM’s beginner’s course is for those at the starting line. It’s a seven-hour course that teaches the basics of AI and ML, in addition to helping you get to grips with the development cycle for a machine learning model. As a primer for the concepts, it’s one of the best AI ML online courses available.
Key Features and Benefits
- Provided by a Fortune 50 company that’s one of the leaders in the AI field
- Created by a Senior Data Scientist who currently works for IBM
- You receive a sharable certificate that looks great on your LinkedIn profile
- No completion of other AI machine learning courses is required to apply
Enrollment Details
“Free” is always a nice price tag to see on anything, and that’s what you get with this course, at least when trialing the course. Enrolment is semi-regular, with batches of students accepted every few months, and you get to reset deadlines based on when you can complete its modules. IBM says the course contains seven hours of content. Your experience may vary depending on how quickly (or otherwise) you adapt to the content.
Course 3 – Post Graduate Program in AI and Machine Learning (Purdue University)
Career Karma ranks this as one of the best AI ML courses online, and it’s hard to argue given that this is a near-year-long course offered with backing from industry professionals at IBM. It’s more bootcamp than formal course, though, so expect to be put through your paces with intensive hackathons and sprints that cover a huge number of AI tools. Combine that with real-world projects (using datasets from companies like Twitter and Uber) and you have a fast-paced and valuable course.
Key Features and Benefits
- Any extremely modern curriculum that takes in real-world examples from tech industry giants
- Backed by IBM to further the real-world experience delivered
- You receive a postgraduate certificate from an established university
- The online bootcamp experience is great for people who prefer fast-paced and intensive learning
Enrollment Details
Enrollment is set for May of each year, with the course lasting for 11 months thereafter. You’ll need to hit some criteria to apply. The course asks for a minimum of a Bachelor’s degree where you’ve obtained at least 50% on your modules, as well as a couple of years of work experience. That work experience requirement may be an issue for people who haven’t started their careers. Still, it’s a cost-effective program, with the course costing £2,990 (approx. €3,400).
Course 4 – Machine Learning Crash Course (Google AI)
If time is of the essence and you just want a crash course in what machine learning is and how it applies to your business, Google provides the answer with this option. At just 15 hours, it’s a course you can complete over an intensive weekend of study. It’ll introduce you to some real-world case studies, with lectures coming directly from industry heads at Google.
Key Features and Benefits
- Contains 25 lessons (with 30 exercises) to expand and test your knowledge
- Get industry insight from Google experts who work in the AI and ML fields
- You don’t have to pay a euro to take part in this course
- Includes interactive visualizations of real-world models that are great for tinkerers
Enrollment Details
Google presumes no prior knowledge of machine learning in this course, though it recommends that you’re comfortable with programming in Python and understand complex statistical concepts. Knowledge of the NumPy library is especially helpful. Assuming you build up a knowledge base (Google offers other courses to cover these foundations), you can enroll at any time and get a free course that you can fit around your schedule.
Additional Resources for AI and ML Learning
Great AI ML courses can teach you the fundamentals and offer direct experience, ideally coming from professionals in the industry. But it’s what you do outside of your formal and certified studies that can make the biggest difference to your career prospects. These additional resources both supplement what you learn from the above courses and allow you to continue developing your skills once you have your shiny new certificate:
- Online forums and communities
- Podcasts and YouTube channels dedicated to machine learning and AI
- Books and eBooks
- Conferences, workshops, and career-centric bootcamps
Use AI & ML Courses Today to Benefit Tomorrow
Consider these facts if you need any more convincing that AI and machine learning courses are right for you. The average machine learning engineer earns between €66,585 and €118,169 per year, with jobs in AI easily climbing into the six-figure range as well. Your career prospects get a boost when you study AI and ML. But remember – a certification alone is not enough.
These are fast-evolving fields, and only those who dedicate themselves to continued learning (and the adaptation that comes with market changes) excel. Start your journey with one of the four courses in this article and then continue down the educational path.
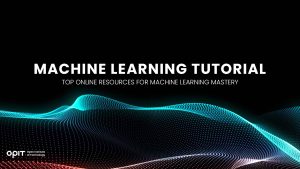
It can often feel like a computer has a “brain,” especially given modern machines’ abilities to run complex calculations and handle instructions. But all of those machines need people behind them to program algorithms and help them to learn based on explicit instructions. That’s where machine learning comes in.
This branch of artificial intelligence brings a machine’s “brain” closer to the real thing than ever before. It’s all about teaching the machine how to do more than simply execute, as machine learning is all about making a machine “think” (based on instructions and algorithms) so it can improve over time. That ability to “think” is crucial in modern business because it gives companies the ability to analyze patterns – both operational and consumer-based – enabling them to make smarter decisions.
But these businesses need people who understand how to create machine learning models. That’s where you come in. With the right machine learning tutorial under your belt, you set yourself up for a career in a field that has only just started to show glimpses of its potential.
The Best Machine Learning Tutorials
Finding the best online tutorial for machine learning isn’t easy given the sheer volume of options available. Analyzing each one based on what it teaches (and how useful it will be to your career) takes time, though you can save yourself that time by checking out the three tutorials highlighted here.
Tutorial 1 – Intro to Machine Learning (Kaggle)
As tempting as it may be to run before you can walk, you need an introduction to the basic concepts of machine learning prior to focusing on more practical applications. Enter Kaggle’s machine learning tutorial. This seven-lesson course takes about three hours of self-guided learning to complete and will leave you with a solid grounding in machine learning that you can take into more industry-focused courses.
The majority of the seven lessons – barring the first – is split into two parts. First comes a tutorial where you’ll learn about the concepts that the lesson introduces, with the second part being an exercise that tests your new skills. Along the way, you’ll learn the basics of how machine learning models work and why you need them to explore large datasets. Other lessons focus on building and validating a model, with the later lessons introducing more complex algorithms, such as random forests, and giving you a chance to test your skills in competitions.
Though this is a beginner-focused tutorial, you’ll need a solid understanding of Python before making a start. Without experience in this programming language, you’ll feel like you’re truly lost in a random forest before you ever get to learn what that term actually means. On the plus side, the tutorial has an active discussion community (which includes the course instructor Dan Becker) that can help you along and point you in the direction of other courses that supplement this one.
Tutorial 2 – Making Developers Awesome at Machine Learning (Machine Learning Mastery)
This machine learning tutorial is less a structured course and more a series of articles and step-by-step instructional lessons that take you from the foundations of machine learning to more advanced concepts. That method of breaking the course into multiple stages is ideal for students of all experience levels. Complete beginners can start with the “Foundations” level and work their way up while those with more experience can dip into specific subjects that give them trouble or will build on their existing skills.
The course is split into four sections – Foundations, Beginner, Intermediate, and Advanced. At the Foundations level, you’ll learn about the statistical concepts and models that underpin machine learning, giving you a solid basis to move into the Python programming taught in the Beginner section. Once you have a grasp of Python, the Intermediate section teaches you about deep learning and how to code machine learning algorithms. By the time you hit the Advanced stage, you’ll be working on complex subjects like computer vision and natural language processing.
With its less structured nature, this tutorial is great for people who want to dip in and out and those who need to hone in on a specific aspect of machine learning. It’s also a good choice for beginners because it covers practically everything you’ll need to know. Unfortunately, the lack of structure means you don’t get an official certification from the tutorial. Some students may also not like the “hub” nature of the tutorial, as it links you to tons of different web pages that can lead to confusion over time.
Tutorial 3 – Machine Learning Crash Course With TensorFlow APIs (Google)
If you already have a mathematical foundation (as well as some basic understanding of machine learning), Google’s tutorial helps you take your skills to the next level. You’ll need to understand algebra, statistics, and basic trigonometry, in addition to having some understanding of Python, to get started. But assuming you have all of that, this machine learning tutorial exposes you to real-world examples of the technology in action.
It’s a 25-lesson course that contains 30 exercises covering topics like model development and testing, data representation, and building neural networks. According to Google, it takes about 15 hours of self-guided study to complete, though your time may vary depending on how much you already know before you start the course.
The biggest advantage of this tutorial is the name attached to it. Google is a major player in the tech industry and the presence of its name on your CV instantly shows employers that you know your stuff. The course material is also delivered by lecturers who work at or for Google, allowing them to bring their real-world experiences into their lessons. On the downside, the tutorial’s prerequisites make it unsuitable for beginners, though Google does offer more basic courses (both in machine learning and Python) to help you build the required foundation.
Factors to Consider When Choosing a Machine Learning Tutorial
The three options presented above all make a solid case for the best online tutorial for machine learning, though each offers something different based on your current skill level. To make the best choice between the three (and any other tutorials you find) you should consider these factors before committing yourself.
Your Current Skill Level
Diving into neural networks before you even know how machine learning works is like trying to row upstream without a paddle. You’re going to get stuck in rough waters and the end result won’t be what you want it to be. Be honest with yourself about your current skill level to ensure you don’t start a tutorial that’s too difficult (or too simple) for your abilities.
Programming Languages
There’s no getting away from the fact that you’ll need to feel comfortable with programming before taking a machine learning tutorial. Specifically, you’re likely to need some knowledge of Python, though how much depends on the course you take. Other languages can help, at least in the sense of ensuring you’re familiar with programming, but you need to check the language the course uses before starting.
Specific Topics
Though the basic idea of building a machine “brain” is simple enough to understand, the machine learning waters run deep. There are tons of topics and potential specializations you could study, and not all are useful for your intended career path. Check what the course covers and ensure those topics align with what you hope to achieve once you’ve completed the tutorial.
Time Commitment
If a tutorial takes an hour or two to complete, you don’t really need to worry about how you’ll fit it around your other commitments. But if it takes you down a machine learning rabbit hole (i.e., the Machine Learning Mastery Course), you need to get serious with scheduling. Figure out how much time you can commit to your course per week and choose a tutorial that fits around your commitments.
The Cost
On the plus side, many machine learning tutorials are available free of charge. But if you’re looking for more official certification, or you want to take a more formal course, you’ll usually have to pay for the privilege. Weigh up the course’s cost against the benefit you get out of the backend.
Tips for Getting the Most Out of a Machine Learning Tutorial
Anybody can start a machine learning tutorial, but only the truly committed will complete and actually get the most out of the materials. Follow these tips to ensure you’re spending your time wisely on the tutorial you choose:
- Set clear goals from the outset that define what you want to achieve with the tutorial and where it’s supposed to lead you.
- Dedicate time to learning every week because regularity is the key to making the information you absorb stick in your mind.
- Engage with any communities related to your tutorial to learn from your peers and ask questions about the tutorial’s content.
- Apply what you learn to real-world problems, either via the course itself or by searching for examples of what you’ve learned being put into action.
- Update your knowledge and skills regularly with further tutorials because what you learn today may be out of date tomorrow.
Find the Best Online Tutorial for Machine Learning for You
There is no single “best” machine learning tutorial on the web because each approaches the subject differently. Some assume you have no knowledge at all and will start with basics before moving you into deeper subjects. Others require you to understand the computing concepts (mathematical and programmatical) that underpin machine learning before you can get started. Understand what the course offers, and what it needs from you, before you get started.
Regardless of your choice, getting started is the most important thing you can do. Once you’ve chosen a tutorial, commit yourself to it fully to take your first step (or potentially a giant leap) into a career that’s only going to grow as machine learning models become more common in business.
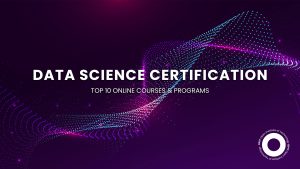
Data science is likely the most sought-after profession today. With top tech organizations looking for talent across the world, this field is highly competitive. That’s why professional improvement represents a crucial aspect of this rapidly-evolving industry.
Getting an approved certificate is the best way to gain the necessary knowledge and a confirmation of your data science skills. This article will give you a list of the 10 best online courses and data science certificate programs that offer worldwide recognized certification.
Factors to Consider When Choosing a Data Science Certification Course
There’s plenty of criteria to look at when choosing a data science certification course online. Of course, the content of the course will be of most interest, especially since data science is a broad field. But several other aspects are also worth researching:
- Program duration
- Flexibility – is it on a fixed timeline or self-paced
- Instructor quality and the reputation of the institution
- Pricing
- Whether the program offers practical projects and hands-on work
- Whether the institution will help you land your next job
Top 10 Online Data Science Certification Courses & Programs
Here’s a brief overview of what the top online courses in data science have to offer. Courses and programs on our list come from respected institutions that hire world-class lecturers and will provide the best certification for data science you could get without setting foot on campus.
Harvard University – Professional Certificate in Data Science
Getting an education in data science from Harvard University is one of the best options in the market. This online course teaches essential skills in programming, modeling, statistics, data visualization, and numerous data science tools.
The Professional Certificate in Data Science course is self-paced and represents an introductory course tailored for beginners who want to advance their skills. You’ll also learn through relevant case studies by analyzing data from real-life examples. The program includes working in the R environment.
The price of this Harvard program is $991, with an available 10% discount. The course runs through the edX platform, and allows you free access to the entire curriculum at your leisure. If you decide for the minimal 2-3 hour weekly commitment, the certificate will take roughly 17 months to complete.
Cloudera – Data Platform Generalist Certification
The Data Platform Generalist test by Cloudera is excellent because it enables learners to take various roles within the data science industry. While the exam focuses on Cloudera’s data platform, the program certifies you as a general data science professional, meaning you can pursue a career in data engineering and analytics, development, administration, and similar fields.
The certification consists of a single 90-minute exam with 60 questions. Cloudera doesn’t state the minimal score needed to pass the exam because the point of the certification program is to do it the best you can rather than aiming for a specific score.
According to the Cloudera website, this certification costs $330. Upon completing the exam, you’ll get a certificate that lasts for two years.
IBM – Data Science Professional Certificate
As one of the industry leaders, IBM provides an exceptional course in data science. The course teaches the basics of data science, focusing on the work methodology via Python and SQL. The Data Science Professional Certificate program helps beginners in the field via hands-on work, with exercises in data set importing, analysis, cleaning, and visualization.
The online certificate course in data science consists of 10 parts. After the first three introductory courses, the following six focus on working in Python, while the final one deals with applied data science. This is a flexible, self-paced program suitable for beginners.
Enrolling in this IBM data science program is free via Coursera, provided you have a monthly subscription. The courses require about three hours of work per week. At that tempo, you should complete the program and receive your data science certification within five months.
Data Science Council of America – Senior Data Scientist
As the name implies, the Data Science Council of America (DASCA) counts among the leading authorities on data science in the U.S. and worldwide. The Senior Data Scientist program enjoys global recognition and takes place entirely online.
This program provides excellent resources that candidates can use to prepare for the exams. Plus, the resources are quality reading for the purposes of professional improvement. The learning material and the program itself are suitable for more experienced learners.
Upon enlisting, you’ll need to cover a one-time fee of $775. Once you receive the resources, you’ll have six months to prepare for the exam. The recommended study time is up to 10 hours weekly.
John Hopkins University – Data Science Specialization
When a reputable institution like the John Hopkins University offers a specialization in data science, there’s no reason to miss that opportunity. Hosted by Coursera, this Data Science Specialization course is built around practical applications of actual data.
The online program provides learners the chance to create a genuine data product. Along with learning, you’ll also be building a respectable portfolio that will come in handy as a demonstration of your newly acquired skills.
Like other Coursera programs, this specialization is also free with a subscription to the service. The program is flexible in terms of time commitment. If you devote an hour a day to it, you can complete the specialization in about 11 months.
Microsoft – Azure AI Fundamentals
Microsoft has proven to be not only a tech giant but an excellent knowledge hub. With Azure AI Fundamentals, this renowned company offers expertly crafted training in the basics of working with artificial intelligence. Through this certification program, learners can gain a thorough understanding of AI and become skilled in the latest technologies.
This online data science certificate course will be suitable even for complete beginners, although a basic level of programming skills would give you an easier start. The program comes in two variants: self-paced and led by a professional instructor.
The program costs only €99 and awards a permanent Microsoft certificate. You can also try out the course with a trial subscription, and there’s an available practice assessment test that will help you understand where you stand before enrolling.
MIT – MicroMasters Program in Statistics and Data Science
If you’re looking for an intensive program that will teach you advanced data science skills, MIT has just the thing. The MicroMasters in Statistics and Data Science is a result of a collaboration between the world-renowned MIT and edX, a trusted learning platform.
This program includes working on data sets from real-world examples, as well as understanding the leading machine learning models. Upon finishing, candidates will be eligible for different titles within the field of data science.
The program consists of five courses and may last up to 14 months with about 14 hours of weekly engagement. The edX platform lists the program price at $1,350.
Open Group – Certified Data Scientist
The Open Group consists of numerous global organizations, with some of the most distinct members being technology giants like IBM, Intel, Fujitsu, and Huawei. The Certified Data Scientist certification that the group provides is a credential recognizes around the globe.
The structure of this program is quite unique. It doesn’t include courses or exams. Instead, applicants need to demonstrate practical data science skills in written form. The point of this certification isn’t to educate, but rather to verify the candidate’s professional capabilities.
The time needed to get the certificate will vary depending on your proficiency level. The certificate is permanent, and Open Group discloses its price via contact.
Stanford University – Machine Learning Certification
Stanford University is home to some of the world’s finest lecturers. The institution provides a machine learning program in collaboration with Coursera and, as a practical, hands-on experience, it’s something eager learners shouldn’t miss.
The Machine Learning Certification is an ideal opportunity for beginners to grasp the intricacies of advanced AI and its applications. The program consists of three courses. By the end of the third course, the applicant should be able to build Python machine learning models from the ground up.
Following Coursera’s standard model, this program is free to enroll into, provided the user has a Coursera subscription. With up to nine hours of work weekly, the program shouldn’t last more than three months.
SAS – Certified AI and Machine Learning Professional
SAS is a certification program that operates globally. It offers a Certified AI and Machine Learning Professional program that’s built for people looking for top practical education in these areas. As the name says, this certification is aimed at future data science professionals.
The program includes five courses after which attendees get permanent certification. Upon registering, learners will receive a full year of access to the complete course material, as well as 70 hours of complimentary software use via cloud.
This program is self-paced, but you have to complete it within one year. The price for one year is €1,295.
Tips for Success in Data Science Certification Courses
Enrolling in a data science course is only a part of the process. To be successful, you’ll need to do your best and employ certain techniques:
- Manage your time effectively. Make sure to commit enough time to progress through the course and meet requested deadlines.
- Start building a network with your peers from day one. Collaborate with people who share your interest in data science so that you can build off of each other.
- Never assume you’ve learned everything there is to know. Data science is evolving constantly, and there’s always new skills to develop and additional knowledge to gain.
- Build a strong portfolio that will increase your chances of finding a job in the field. The best data science certification programs represent an ideal start.
Get Certified in One of the Top Professions Today
Getting a data science certificate online can open up a career path in a top-paid profession that continues to grow. With certification from one of the leading institutions in the field, you’ll be on the right track to success.
Our list contains programs and courses from renowned organizations like Harvard, IBM, MIT, and Microsoft. The quality of lecturers is unquestionable, and the programs offer the most up-to-date courses. Whichever certification you choose, you can rest assured you’ll be the best data science certification online.
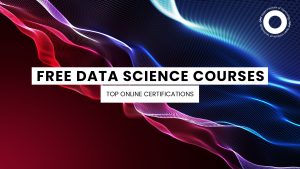
In a world of Big Data, companies need people who have the ability to analyze and reach conclusions from the reams of data they collect about customers. But data science extends far beyond the corporate. Any industry that uses data (i.e., practically all of them) needs data-minded people who can use the latest AI-driven tools to help them scour large datasets.
That’s where you come in. As a potential data scientist, you’ll enter an industry that’s experiencing enormous growth to the point where it will be worth $103 billion (approx. €96.37 billion) by 2027. That market growth translates into demand for talented data scientists, which is already seen today as Coresignal’s data – 8,000 available job postings across eight leading positions in the first five months of 2022 alone – demonstrates.
So, the benefits of earning a free data science certification are obvious – you’re entering a growing industry with huge demand that leads to a better salary. But you need to know which courses will help you break into that industry. This article highlights four of the best free data science courses around.
Top Four Free Data Science Courses
As wonderful as the word “free” may be to budget-conscious students, you still need to know that you’re getting something of value from your data science course. The following options deliver a stellar educational experience and leave you with a qualification that employers recognize.
An Introduction to Data Science (Udemy)
Every journey starts with a first step, and it’s crucial that you take the first step into data science with a course that covers the basics and lays a foundation on which you can build. An Introduction to Data Science does just that by teaching you what data science is and how it applies to the modern world.
That teaching starts with a history lesson that shows how interactions with data (and data collection methods) have evolved over the years. From there, you’ll learn how data science applies in modern industry and discover the difference between actual valuable data and the oodles of “noise” that are in datasets.
It’s a quick and easy course, weighing in at 43 minutes spread across six video lectures, so you don’t have to make a huge time commitment. It’s delivered online by a Google Certified Python Expert named Kumar Rajmani Bapat and is ideal for getting the basics of data science down before you move on to a more intensive or focused course.
But the focus on the basics is also the biggest issue with this course. Rather than showing you the techniques a data scientist uses, the course focuses on what data science is and offers a roadmap for getting into the industry. It’s more about “what” than “how,” which may make the course too rudimentary for people who already have some knowledge of the subject. It’s also worth noting that this isn’t one of those free data science courses with certificate, as you’ll need to pay for an Udemy subscription to get your hands on a certificate of completion. You can still watch the videos and complete the course for free, though.
Introduction to Data Science (SkillUP)
With a similar name to the above Udemy course, you’d be forgiven for assuming that SkillUP’s Introduction to Data Science program teaches the same stuff. Though the course is aimed squarely at beginners, it takes a more in-depth approach that makes it the ideal follow-up to Udemy’s offering.
You start with the basic spiel about what data science is and how it applies to modern industry. But from there, the course tips into actual application by demonstrating some of the best Python programming libraries to use in the field. You’ll also dig deep into the algorithms used in data science, with linear regression analysis, confusion matrices, and logistic regression all getting some time to shine.
Given this higher focus on the skills you’ll need to learn to become a data scientist, the course is longer than Udemy’s offering. It clocks in at seven hours of videos and tutorials, all of which you access online and work through at your own pace. The course also expects you to have a solid grasp of math and programming (some experience with Python is a must) so this isn’t ideal for complete beginners to computer science.
This is a data science free online course with certificate, though there is a caveat. SkillUP only provides 90 days of free access to the course. If you feel it will take longer than that to get through the seven hours of tutorials, you’ll need to enroll in a paid subscription. The best approach here is to only start the course when you’re confident that you can block out the time needed to wrap it up within 90 days.
IBM Data Science Professional Certificate (Coursera)
Aimed squarely at the career-focused individual, IBM’s data science course is all about building the skills that set you on the right path to a career in the field. It takes a more practical approach, starting you off with the fundamentals before pushing you into a project where you’ll work with a real-world dataset and publish a report that’s analyzed by stakeholders simulating what you’ll experience in the working world.
The good news is that you don’t need to know anything about data science to get started with the course. It holds your hand as you learn the basics of what data science is (including what a data scientist actually does) and teaches you about the tools and programming languages you’ll use in the field. Once you have a grasp on the fundamentals, you’ll learn how to analyze and visualize data, in addition to creating machine learning models using Python, before wrapping up with the previously mentioned project.
The IBM Data Science Professional Certificate is a more intensive course than the others on this list. It’s essentially a mini degree, requiring you to invest 10 hours per week for five months into your learning. However, the course is provided entirely online, allowing you to schedule that learning time as you see fit. You’ll work through 10 modules as part of the certificate.
That time commitment may be a downside for those who can’t put 10 hours per week into a course, though that downside is outweighed heavily by the fact that you come out with an IBM certification. Having one of the leading names in computing on your certificate is enough to make any employer sit up and take notice.
Data Analysis With Python (freeCodeCamp)
The Python programming language (along with SQL and a few others) underpins almost everything that the modern data scientist does. Data Analysis with Python takes that concept and runs with it by providing a course that digs into using Python to read, analyze, and visualize data.
Along the way, you’ll learn about the basics of both Python and data analysis, though the real highlight comes from the many libraries and tools the course introduces. You’ll use Seaborn, Numpy, Mayplotlib, and Pandas during the course. All of which are libraries used by professionals to extract and visualize data. The course wraps up with a series of five projects, each testing a different set of skills learned via the modules, with your certification coming after you’ve completed all five.
This is one of those free data science courses that’s entirely self-paced and there are no time constraints or commitments involved. Once you’ve signed up for freeCodeCamp, you can save your progress through the course at any point and return whenever you’re ready. Theoretically, this means you could start the course, save your progress, and then return to it months later, though that isn’t recommended if you want to keep the information fresh in your mind. All told, the course contains 37 modules, plus the five projects required for certification, making it one of the most in-depth Python courses around.
The focus on Python is great for those who are unfamiliar with the language, though it also creates some issues. Namely, this isn’t the right course for those who don’t understand data science fundamentals. It jumps straight into analyzing datasets using Python, so those who don’t really understand what datasets are or how they apply to the modern world should start with a more beginner-oriented course.
Tips for Choosing the Right Data Science Course
You get the same benefit from all of the listed data science online courses – free entry. But each course offers something different. Use these tips to determine which is the right choice for you:
- Assess your current skill level to pick a course that delivers what you need to know right now rather than a course that forces you to run before you can walk.
- Determine your learning goals so you can see how the course fits into your roadmap for becoming a data scientist.
- Consider the course’s format and duration as both will play a huge role in how you schedule your learning around your other commitments, be they work-related or personal.
- Look for courses that offer hands-on project work once you’ve moved beyond learning the basics of data science.
- Read reviews and testimonials from other students to see if people in your position get actual value from the course.
Start Your Journey With Free Data Science Courses Online
Every journey starts with a first step, and that first step could take you into a career that has massive potential for growth if you opt for the data science path. The four courses listed here each offer something different, from exploring the basics of what data science is to digging deep into the programming tools you’ll use to conduct data analysis and visualization. Completing one of the four sets you on the right path, though completing all four gives you a solid grounding (and a set of certifications) that make you immensely attractive to employers.
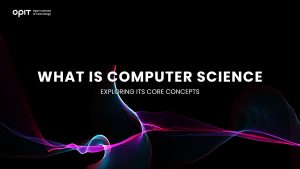
Large portions of modern life revolve around computers. Many of us start the day by booting a PC and we spend the rest of our time carrying miniaturized computer devices around – our smartphones.
Such devices rely on complex software environments and programs to meet our personal and professional needs. And computer science deals with precisely that.
The job of a computer scientist revolves around software, including theoretical advances, software model design, and the development of new apps. It’s a profession that requires profound knowledge of algorithms, AI, cybersecurity, mathematical analysis, databases, and much more.
In essence, computer science is in the background of everything related to modern digital technologies. Computer scientists solve problems and advance the capabilities of technologies that nearly all industries utilize.
In fact, this scientific field is so broad that explaining what is computer science requires more than a mere definition. That’s why this article will go into considerable detail on the subject to flesh out the meaning behind one of the most important professions of our time.
History of Computer Science
The early history of computer science is a fascinating subject. On the one hand, the mechanics and mathematics that would form the core disciplines of computer science far predate the digital age. On the other hand, the modern iteration of computer science didn’t start until about two decades after the first digital computer came into being.
When examining the roots of computer science, we can go as far back as the antiquity era. Mechanical calculation tools and advanced mathematical algorithms date back millennia. However, those roots are too loosely connected to computer science.
The first people who started exploring the foundations of what is computer science today were Wilhelm Schickard and Gottfried Leibniz in early and late 17th century, respectively.
Schickard is responsible for the design of the world’s first genuine mechanical calculator. Leibniz is the inventor of a calculator that worked in the binary system, the universally known “1-0” number system that paved the way for the digital age.
Despite the early advances in the mentioned fields, it would be another 150 years after Leibniz before mechanical and automated computing machines saw industrial production. Yet, those machines weren’t used for any other purpose apart from calculations.
Computers became more powerful only in the 20th century. Like many other technologies, this branch saw rapid development during the last one hundred years, with IBM creating the first computing lab in 1945.
Yet, while plenty of research was happening, computer science wasn’t established as an independent discipline. That would take place only during the 1960s.
Early Developments
As mentioned, the invention of the binary system could be considered a root of computer science. This isn’t only due to the revolutionary mathematical model – it’s also because the binary number system lends itself particularly well to electronics.
The rise of electrical engineering moved forward inventions like the electrical circuit, the transistor, and powerful data storage solutions. This progress gave birth to the earliest electrical computers, which mostly found use in data processing.
It didn’t take long for massive companies to start using the early computers for information storage. Naturally, this use made further development of the technology necessary. The 1930s saw crucial milestones in computer theory, including the groundbreaking computational model by Alan Turing.
Not long after Turing, John von Neumann created a model of a computer that can store programs. By the 1950s, computers were in use in complex calculations and data processing on a large scale.
The rising demand made the binary machine language too unreliable and impractical. The successor, the so-called assembly language, soon proved just as lacking. By the end of the decade, the world saw the first program languages, which soon became the famed FORTRAN (Formula Translation) and COBOL (Common Business Oriented Language).
The following decade, it became obvious that computer science is a field of study in itself, rather than a subset of mathematical or physical disciplines.
Evolution of Computer Science Over Time
As technology kept progressing, computer science needed to keep up. The first computer operating systems came about in the 1960s, while the next two decades brought about an intense expansion in graphics and affordable hardware.
The combination of these factors (OS, accessible hardware, and graphical development) led to advanced user interfaces, championed by industry giants like Apple and Microsoft.
In parallel to these discoveries, computer networks were advancing, too. The birth of the internet added even more moving parts to the already vast field of computer science, including the first search engines that utilized advanced algorithms, albeit not at the same level as today’s engines.
Furthermore, greater computational capabilities created a need for better storage systems. This included larger databases and faster processing.
Today, computer science explores all of the mentioned facets of computer technology, alongside other fields like robotics and artificial intelligence.
Key Areas of Study in Computer Science
As you’ve undoubtedly noticed, computer science grew in scope with the development of computational technologies. That’s why it’s no surprise that computer science today encompasses many areas that deal with every aspect of the technology currently imaginable.
To answer the question of what is computer science, we’ll list some of the key areas of this discipline:
- Algorithms and data structures
- Programming languages and compilers
- Computer architecture and organization
- Operating systems
- Networking and communication
- Databases and information retrieval
- Artificial intelligence and machine learning
- Human-computer interaction
- Software engineering
- Computer graphics and visualization
As is apparent, these areas correspond with the historical advances in computational technology. We’ve talked about how algorithms predate the modern age by quite a lot. These mathematical achievements brought about early machine languages, which turned into programming languages.
The progress in data storage and the increased scope of the machines resulted in a need for more robust architecture, which necessitated the creation of operating systems. As computer systems started communicating with each other, better networking became vital.
Work on information retrieval and database management resulted from both individual computer use and a greater reliance on networking. Naturally, it didn’t take long for scientists to start considering how the machines could do even more work individually, which marked the starting point for modern AI.
Throughout its history, computer science developed new disciplines out of the need to solve existing problems and come up with novel solutions. When we consider all that progress, it’s clear that the practical applications of computer science grew alongside the technology itself.
Applications of Computer Science
Computer science is applied in numerous fields and industries. Currently, computer science contributes to the world through innovation and technological development. And as computer systems become more advanced, they are capable of resolving complex issues within some of the most important industries of our age.
Technology and Innovation
In terms of technology and innovation, computer science finds application in the fields of graphics, visualization, sound and video processing, mathematical modeling, analytics, and more.
Graphical rendering helps us visualize concepts that would otherwise be hard to grasp. Technologies like VR and AR expand the way we communicate, while 3D models flesh out future projects in staggering detail.
Sound and video processing capabilities of modern systems continue to revolutionize telecommunications. And, of course, mathematical modeling and analytics expand the possibilities of various systems, from physics to finance.
Problem-Solving in Various Industries
When it comes to the application of computer science in particular industries, this field of study contributes to better quality of life by tackling the most challenging problems in key areas:
- Healthcare
- Finance
- Education
- Entertainment
- Transportation
Granted, these aren’t the only areas where computer science helps overcome issues and previous limitations.
In healthcare, computer systems can produce and analyze medical images, assisting medical experts in diagnosis and patient treatment. Furthermore, branches of computer science like psychoinformatics use digital technologies for a better understanding of psychological traits.
In terms of finance, data gathering and processing is critical for massive financial systems. Additionally, automation and networking make transactions easier and safer.
When it comes to education and entertainment, computer science offers solutions in terms of more comprehensible presentation, as well as more immersive experiences. Many schools worldwide use digital teaching tools today, helping students grasp complex subjects with fewer obstacles compared to traditional methods.
Careers in Computer Science
As should be expected, computer science provides numerous job opportunities in the modern market. Some of the most prominent roles in computer science include systems analysts, programmers, computer research scientists, database administrators, software developers, support specialists, cybersecurity specialists, and network administrators.
The mentioned roles require a level of proficiency in the appropriate field of computer science. Luckily, computer science skills are easier to learn today – mostly thanks to the development of computer science.
An online BSc or MSc in computer science can be an excellent way to get prepared for a career in the most sought-after profession in the modern world.
On that note, not all computer science jobs are projected to grow at the same rate by the end of this decade. Profiles that will likely stay in high demand include:
- Security Analyst
- Software Developer
- Research Scientist
- Database Administrator
Start Learning About Computer Science
Computer science represents a fascinating field that grows with the technology and, in some sense, fuels its own development. This vital branch of science has roots in ancient mathematical principles as well as the latest advances like machine learning and AI.
There are few fields worth exploring more today than computer science. Besides understanding our world better, learning more about computer science can open up incredible career paths and provide an opportunity to contribute to resolving some of the burning issues of our time.
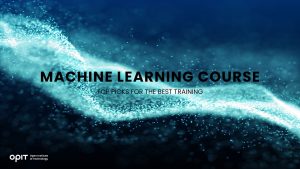
Data permeates almost every aspect of our lives. Trying to make sense of it all is a Herculean endeavor that would take humans years (if not centuries). But fear not; it’s machine learning to the rescue.
Machine learning algorithms can comb through data in a matter of days or even hours, uncovering valuable insights. Many industries have already experienced numerous benefits of these algorithms, yet the field promises to get even bigger and better.
However, we shouldn’t discard humans just yet. They still play an essential role in this process.
Machine learning algorithms couldn’t parse and interpret data correctly without human guidance. As the machine learning field grows, so will the need for skilled data scientists.
One way to acquire the skills necessary to participate in this game-changing field is by taking a machine learning course. When chosen wisely, this course will provide you with crucial theory and invaluable practice to enter the field with a bang or take your knowledge to the next level.
To ensure you choose the best machine learning course, we’ve compiled a list of our top five online picks.
Factors to Consider When Choosing a Machine Learning Course
Just like data, there are tons of courses online. Taking all of them would not be humanly possible. And frankly, not all of these courses would be worth your time. Remember these factors when browsing online learning platforms, and you’ll pick the best machine learning course each time.
Course Content and Curriculum
Shakespeare once said, “Expectation is the root of all heartache.” Believe it or not, this quote will benefit you immensely when choosing an online machine learning course.
Just because a course is named Machine Learning, it doesn’t mean it will be helpful to you. The only way to ensure the course is worth taking is to check its curriculum. Provided the description isn’t misleading, you’ll immediately know whether the course suits your educational and professional needs.
Instructor’s Expertise and Experience
Who teaches the course is as important as what is taught (if not more). Otherwise, you could just pick up a book on machine learning with the same content and try to make sense of it.
So, when a machine learning course piques your interest, check out the instructor.
Are they considered an authority in machine learning? Are they industry veterans?
A quick Google search will tell you all you need to know.
Course Duration and Flexibility
“Can I fully commit to this course?” That is the question to ask yourself before starting a machine learning course.
One look at the course’s description will tell you whether it takes an hour or months to complete. Also, you’ll immediately know if it is self-paced or fixed-timeline.
Hands-On Projects and Real-World Applications
No one can deny the value of theoretical knowledge in a machine learning course. There’s no moving on without understanding machine learning algorithms and underlying principles.
But how will you learn to use those theoretical concepts in practice? That’s right, through hands-on projects and case studies.
Ideally, your chosen course will strike the perfect balance between the two.
Course Reviews and Ratings
Sure, it’s easy to manipulate reviews and ratings. But it’s even easier to spot the fake ones. So, give the rating page a quick read-through, and you should be able to tell if the course is any good.
Certification and Accreditation
Certified and accredited courses are a must for those serious about a career in machine learning. Of course, these courses are rarely free. But if they help you land your dream job, the investment will be well worth it.
Top Picks for the Best Machine Learning Courses
We’ve also considered the above-mentioned factors when choosing our top picks for online machine learning courses. Without further ado, check out the best ones to help you learn or improve machine learning skills.
Supervised Machine Learning: Regression and Classification
This course has a lot of things going for it. It was one of the courses that popularized the entire concept of massive open online courses. And it is taught by none other than Andrew Ng, a pioneer and a visionary leader in machine learning and artificial intelligence (AI). In other words, this course is the gold standard by which every machine learning course is evaluated.
Here are all the important details at a glance:
- The course is beginner-friendly and features flexible deadlines.
- It lasts 11 weeks, each covering different machine learning techniques and models (six hours per week).
- It covers the fundamentals of machine learning and teaches you how to apply them.
- The skills you will gain include regularization to avoid overfitting, gradient descent, supervised learning, and linear regression.
- You’ll earn a certificate after completing the course.
The only thing to note about the certificate is that you must sign up for a Coursera membership ($39/€36 a month) to receive it. Otherwise, you can audit the course for free. To apply, you only need to create a Coursera account and press the “Enroll” button.
Machine Learning With Python
Another fan-favorite on Coursera, this machine learning course uses Python (SciPy and scikit-learn libraries). It’s offered by IBM, a company at the forefront of machine learning and AI research.
Here’s what you need to know about this course:
- The course is beginner-friendly but requires a great deal of calculus knowledge.
- It’s divided into four weeks, each dedicated to one broad machine learning task (regression, clustering, classification, and their implementation).
- By the end of the course, you’ll learn the theoretical fundamentals and numerous real-world applications of machine learning.
- The emphasis is placed on hands-on learning.
- A certificate is available, provided you apply for a Coursera membership ($39/€36 a month).
A Coursera account is all you need to apply for this course. You can start with a 7-day free trial. You’ll have to pay $39 (approximately €36) a month to continue learning.
Machine Learning Crash Course
Google’s Machine Learning Crash Course is ideal for those who want a fast-paced approach to learning machine learning. This intensive course uses TensorFlow, Google’s popular open-source machine learning framework.
Check out these facts to determine whether this is the best machine learning course for you:
- You can take this course as a beginner if you read some additional resources before starting.
- The course consists of 25 lessons that you can complete in 15 hours.
- Google researchers present the lessons.
- It perfectly combines theoretical video lectures (machine learning concepts and engineering), real-world case studies, and hands-on exercises.
- No certificate is issued upon completion.
Enrolling in this course is pretty straightforward – just click the “Start Crash Course” button. The course is free of charge.
Machine Learning A-Z: Hands-On Python & R in Data Science
As its name implies, this Udemy course is pretty comprehensive. Two data scientists teach it, primarily focusing on practical experiences (learning to create machine learning algorithms). If you feel like you’re missing hands-on experience in machine learning, this is the course for you.
Before applying, consider the following information:
- The course can be beginner-friendly, provided you have solid mathematics knowledge.
- It consists of video lessons and practical exercises (around 40 hours total).
- The introductory portion focuses on regression, classification, and clustering models.
- You’ll receive a certificate of completion.
To gain lifetime access to this course, you’ll need to pay $89.99 (a little over €83). Applying for it is a matter of creating an Udemy account and purchasing the course.
Machine Learning Specialization
This advanced course is the course you want to take when mastering your knowledge of machine learning. Or perhaps we should say courses since this specialization consists of six separate courses. The program was created by Andrew Ng, who also serves as an instructor (one of four total).
Here’s a quick overview of the course’s key features:
- The course isn’t beginner-friendly; it’s intermediate level and requires previous experience.
- At a pace of three hours per week, it takes approximately seven months to complete.
- The course focuses on numerous practical skills, including Python programming, linear regression, and decision trees.
- Each course includes a hands-on project.
- You’re awarded a shareable certificate upon completion of each course in the specialization.
To begin this challenging yet rewarding journey, create a Coursera account and enroll in the specialization. Then, you can choose the first course—the entire specialization costs around $350 (close to €324).
Additional Resources for Learning Machine Learning
The more you immerse yourself in machine learning, the faster you advance. So, besides attending a machine learning course, consider exploring additional learning resources, such as:
- Books and e-books. Books on machine learning provide in-depth explanations of the topic. So, if you feel that a course’s content is insufficient, this is the path for you. Check out “Introduction to Statistical Learning” (theory-focused) and “Hands-On Machine Learning With Scikit-Learn and TensorFlow.”
- Online tutorials and blogs. Due to the complexity of the field, only a few bloggers post consistently on the topic. Still, blogs like Christopher Olah and Machine Learning Mastery are updated relatively frequently and contain plenty of fascinating information.
- Podcasts and YouTube channels. Keep up with the latest news in machine learning with podcasts like “This Week in Machine Learning and AI.” YouTube channels like Stanford Online also offer a treasure trove of valuable information on the topic.
- Networking and community involvement. You can learn much about machine learning by sharing insights and ideas with like-minded individuals. Connect with the machine learning community through courses or conferences (AI & Big Data Expo World Series, MLconf).
Master Machine Learning to Transform Your Future
An online machine learning course allows you to learn directly from the best of the best, whether individuals like Andrew Ng or prominent organizations like Google and IBM. Once you start this exciting journey, you probably won’t want to stop. And considering all the career prospects machine learning can bring, why would you?
If you see a future in computer science, consider pursuing a degree from the Open Institute of Technology. Besides machine learning, you’ll acquire all the necessary skills to succeed in this ever-evolving and lucrative field.
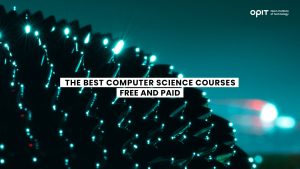
As we continue the slow march towards an AI-powered future, the coding and technical skills computer science graduates have are in increasingly high demand. This demand accounts for the high salary expectations of a computer science expert (the average salary in the field is €5,700 in Germany) and makes you more attractive as a hiring prospect to employers.
The challenge – finding a quality computer science course that provides the knowledge you need and has a reputation that forces employers to take notice. The four courses in this article (combined with related studies) transform you from computing enthusiast to sought-after computer scientist.
Top Free Computer Science Courses
Kicking off this computer science course list, we have a pair of the best free online computer science courses for building a foundation within the subject area.
Course 1 – CS50: Introduction to Computer Science (Harvard University)
If you’re looking for a free course that carries plenty of prestige, anything with the “Harvard” label attached is a good start. CS50 is a self-paced course, with Harvard estimating an 11-week completion time with between 10 and 20 hours of daily studying. It’s offered in English (sadly, no alternative languages exist at the time of writing) and it’s free to take, though you’ll pay $189 (approx. €175) for an official certificate.
The course covers programming language basics, starting with simple web-based HTML and advancing into Python and C. Advanced computing concepts, such as data structures and cybersecurity, are also covered, though you’re getting more of a baseline knowledge than specialized teaching. Think of the course as a computer science primer designed to give you a foundation that’s ideal for moving on to more complex studies. Add to that the Harvard seal of approval, which looks great on any CV, and you have a course that’s available globally and ideal for impressing employers.
Course 2 – CompTIA A+ (CompTIA)
This free course is A+ by name and mostly A+ by nature, with CompTIA advertising it as the perfect pathway to follow if you want a career in IT or computer sciences. You get an industry-recognized credential that employers will love, with the course focusing as much on practical skills (such as thinking on your feet in an IT crisis) as it does on theoretical instruction.
That’s not to say that theory isn’t covered. Once you’ve gotten to grips with the basics of the hardware and various operating systems, you’ll move into practical modules focused on networking, software, and cybersecurity. The course providers carry some industry weight, too, as titans like Dell, HP, and Intel recommend CompTIA’s courses for anybody who wants to break into the workforce.
There are some downsides – namely the minimal theoretical teaching makes it harder to understand why the practical things you’ll learn work. But as a companion piece to a more technical course (such as CS50), A+ is a great way to develop much-needed skills.
Other Notable Free Courses
The two courses listed above are far from the only free computer science course options available, with the following also being solid choices:
- Python for Everybody – Coming from the University of Michigan, this course teaches the ins and outs of a popular programming language used in AI and machine learning.
- IBM Data Science Professional Certificate – As something of a computer science-adjacent course, IBM’s certificate hones in on data science topics, such as visualization and machine learning models.
- Introduction to Computer Science and Programming – Put together by the best minds at the Massachusetts Institute of Technology (MIT), this is a great course for beginners who are starting from square one when it comes to programming.
Top Paid Computer Science Courses
If you have some money to spend on your education (or access to student funding) these are the best courses for computer science students who value a more traditional paid education.
Course 1 – Bachelor in Modern Computer Science (OPIT)
As an entirely online course, OPIT’s offering allows you to learn at mostly your own pace, though you’re still expected to complete coursework and pass exams at appropriate times. It’s a three-year course (though two-year fast-track options are available) and it’s provided by an institution that has European Qualification Framework (EQF) credentials.
Granted, the course doesn’t come cheap, with its €3,600 per year tuition fees adding up to €10,800 for a three-year course. But that money buys you a comprehensive computer science education, starting with the basics of software development before moving on to modern concepts, like AI and cloud computing. Along the way, you’ll earn professional certifications from Microsoft and Google, giving you something tangible to place on your CV even as you’re still studying. Credit transfer is also available for students who started a computer science course elsewhere and want to transfer to OPIT’s offering.
Course 2 – Computational Science and Engineering (Technical University of Munich)
Perpetually hovering around the top 50 universities in the world (it ranked 50th in 2021), the Technical University of Munich (TUM) is like the MIT of Europe. With this course, TUM offers something for students who’ve already started on the computer science track and now feel ready to bring those skills together with applied math and engineering for a Master’s certification.
Lasting four semesters of full-time study, the course costs €152.30 and delivers 120 ECTS credits. You’ll hone in on numerical simulation, focusing on how to develop math-based problem-solving methods that help in developing systems and simulations. Theory is king in this course. But you’ll come away with such a solid grounding in that theory (as well as experience with simulated applications) that prepare you for a computer science and engineering career.
Other Notable Paid Courses
More thought goes into choosing a paid computer science course because you’re investing more than just time into your studies. If neither of the above two courses whets your appetite, the following are a few other notable providers offering courses to European and international students:
- Computer Science BSc by Cambridge University – You get more than a degree from one of the UK’s most prestigious universities with this course. Given that Cambridge University lies in the heart of “Silicon Fen,” this course puts you in the ideal location to gain exposure to over 1,000 Cambridge-based tech companies.
- Computational Thinking for Problem Solving – Devised by the Penn University faculty, this four-week online course starts by teaching the “pillars” of computational thinking, ending with an applied task using the Python programming language.
- Computer Science 101L Master the Theory Behind Programming – Available via Udemy, this course costs about €69 or is available with a monthly subscription to Udemy. It features nearly 12 hours of recorded teaching sessions, alongside articles and other resources, that teach the basics of computer science.
Related Courses for a Well-Rounded Computer Science Education
The courses covered so far focus on computer science, with some variance in a few cases, which is like building the foundations for a house. To turn those foundations into something special (and something from which you can make a living), you may need a few more materials. Computer science-related courses give you those materials, with the following areas being great targets for further study.
Programming Languages
Programming is the beating heart of computer science. Every piece of software you’ll ever use has a program behind it. Most basic computer science courses teach general programming skills, often in Python, but further study into languages like SQL, Java, and C broadens your skillset to make you more attractive to employers.
Web Development
According to web3.career, the average European web developer picks up €70,000 per year, with potential to hit six figures with the right company and training. Many of the basics of web development are things you’ll pick up in a computer science course, though those looking for more formal certification should consider the following:
- Full-Stack Web Development for Free (CodingNinjas)
- Intro to HTML 5 (University of Michigan)
- Web Developer on Google Digital Garage (Google)
Cybersecurity
The European Council’s research suggests that the cost of cybercrimes amounted to €5.5 trillion on the continent alone, with ransomware attacks being among the biggest threats facing EU companies. Therein lies an opportunity – businesses don’t want to lose trillions of euros and your cybersecurity skills could be the shield they need to fend off cyberattacks.
Top cybersecurity courses to consider include:
- Google Cybersecurity (Google)
- The Complete Cyber Security Course (Udemy)
- Introduction to Cybersecurity Foundations (Infosec)
Data Science
Estimates state that the data science industry will have a 29% compound annual growth rate (CAGR) between 2022 and 2029, making it an ever-growing monolith in the computer science sector. Your ability to extract insights from massive datasets could be useful to employers and is buoyed by the following top courses:
- Data Science MicroMasters (University of California San Diego)
- CS109 Data Science (Harvard University)
- Master of Science in Machine Learning and Data Science (Imperial College London)
Tips for Choosing the Right Computer Science Course
The computer sciences courses covered in this article run the gamut from beginner-level programs to full Master’s degrees. If you feel like you’re struggling to navigate the sheer volume of options available, these tips help you pick an appropriate course:
- Be honest with yourself about your current skill level to choose a computer science course that challenges without being overwhelming.
- Compare the course’s curriculum and learning outcomes with your goals to ensure you’ll get what you need from your studies.
- Measure your time commitments (and how the course format allows for these commitments) against those the course demands.
- Research the instructors who created the course and check online reviews from past and current students.
- Determine whether the cost of the course (both monetary and time-wise) delivers a suitable return on your investment.
Start Your Computer Science Journey With the Right Course
Options abound when you’re looking for a computer science course, with quality free options sitting right alongside traditional paid courses. Whatever course you choose, always remember – one step in the right direction still means that you’re moving forward. By choosing a course, you take your first step into a constantly evolving and expanding world that could provide you with a lifelong career.
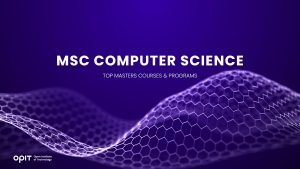
Finding an industry or even area of life that doesn’t utilize digital technologies is quite a challenge today. As computers continue to impact the ways we do business and live, understanding their capabilities and limitations becomes essential. This is the gist of what computer science is all about.
The tasks of computer science keep growing in scope and complexity. This means the demand for professionals in the field is always on the rise. Global companies are always on the lookout not only for people who know computer science but are also experts in the field.
For these reasons, getting an MSc in Computer Science can be the best career move in the modern landscape. Masters in Computer Science allows you to gain detailed knowledge and choose a specialized path. Better yet, holding such a degree elevates your chances of landing a well-paid job at a respectable organization.
Getting an MSc Computer Science is undoubtedly a good idea. You can even do it online, with all of the conveniences of remote learning. Let’s look at the best Masters in Computer Science courses and find out what they offer in terms of professional development.
Factors to Consider When Choosing an MSc Computer Science Program
Picking the right course may be something of a challenge. Numerous institutions offer quality programs, so you might not know where to start or what to look for when making the decision. Here are the key factors that should influence your choice.
Firstly, the reputation of the institution providing the course will matter greatly. Leading universities and learning organizations will offer the most comprehensive programs. Plus, their degrees will be accredited and recognized worldwide.
Next, you’ll need to choose a particular curriculum and specialization that fit your needs and interests. Computer science is a broad field of study, so picking the right study path will be necessary.
The institution you enroll in should have quality faculty members. This aspect is relatively straightforward: If you pick a reputable university, chances are the faculty will be up to par. On a similar note, such institutions will provide ample research opportunities.
The financial aspect is, of course, another important factor. Tuition fees differ considerably between institutions, and some may provide considerable aid for upcoming students. Yet, that doesn’t mean you should opt for the most affordable variant – the combination of a reasonable price and quality education will be the winning one.
When studying on-campus, the location and facilities will be crucial. While not the deciding factor, this may be a tipping point when comparing two otherwise evenly matched institutions.
Lastly, career support is one of the most important advantages you can get from an MSc program. Some institutions provide considerable opportunities for career development, connecting students with leading companies in the field. Additionally, network-building options will matter in this regard.
Top MSc Computer Science Courses and Programs
Norwegian University of Science and Technology
- Location: Gjøvik, Norway
- Duration: Two years
- Study Mode: Full-time
- Requirements: Informatics bachelor’s or engineering degree; minimum average grade: C; minimum informatics credits: 80; documented informatics and mathematics knowledge
- Tuition fees: No fees
- Scholarships/Financial aid: Free program – no financial aid needed
- Career prospects: Machine learning, gaming industry, AI, VR; possibility of Ph.D. program application
Check out MSc in Computer Science at the Norwegian University of Science and Technology.
KHT
- Location: Stockholm, Sweden
- Duration: Two years
- Study Mode: Full-time
- Requirements: Bachelor’s degree from a Swedish or another recognized university in informatics, computer science, or mathematics (minimum 180 ECTS credits); proficient use of the English language – IELTS 6.5, TOEFL 20, PTE 62, ESOL C1 (minimum 180 points)
- Tuition fees: SEK 310,000; application fee is SEK 900
- Scholarships/Financial aid: Scholarships are available from KTH, the Swedish Institute, and associated organizations; full and one-year scholarships available
- Career prospects: Graduates from KHT have moved forward to Ph.D. studies worldwide or found jobs at leading tech companies like Google, Oracle, Saab, Spotify, and Bloomberg.
Check out MSc in Computer Science at KHT.
University Leiden
- Location: Leiden, Netherlands
- Duration: Two years
- Study Mode: Full-time
- Requirements: Bachelor’s degree in AI, Bioinformatics, Computer Science or a related program; English proficiency – IELTS 6.5, TOEFL 90
- Tuition fees: Students from the EU, Suriname, or Switzerland: €2,314 yearly; other students: €19,600 yearly
- Scholarships/Financial aid: Various scholarships available; EU students under the age of 30 are eligible for a Dutch government loan
- Career prospects: Careers in AI, computer science and education, data science, and advanced computer systems
Check out MSc in Computer Science at University Leiden.
Specializations Within MSc Computer Science
Computer science has numerous subcategories and fields of study. These fields are widely different, so you’ll need to choose your specialization carefully. Let’s look at the key disciplines of computer science that you can specialize in and what those disciplines mean.
Artificial Intelligence and Machine Learning
As a field of computer science, AI deals with methods and technologies that allow machines to simulate human intelligence. This includes machine learning, deep learning, and similar disciplines. Through learning methods, either assisted or unassisted by humans, machines can process data and draw conclusions somewhat independently.
Data Science and Big Data Analysis
Data science, as the name implies, deals with data gathering, processing, and analysis. This facet of computer science is particularly important, as it finds plenty of practical applications in business, other sciences, demographics, and statistics.
A subset of data science, big data analysis focuses on extracting information from massive databases. A data scientist’s job is to compile the data and use advanced technological solutions to draw meaningful conclusions. The volumes of data analyzed this way far surpass anything that humans can achieve without computer assistance.
Cybersecurity and Information Security
Today, cybersecurity counts among the most important facets of computer science. Other disciplines gather, produce, and store copious amounts of data which often contain sensitive information. Unfortunately, modern criminals prey on that information to gain access to financial accounts, steal confidential data, and blackmail businesses and individuals.
Cybersecurity attempts to foil attacks from malicious parties. As the methods of crime evolve, so do the technologies meant to fight them. From phishing prevention to protection from hacking, cybersecurity, and information security ensures sensitive data doesn’t end up in the wrong hands.
Software Engineering and Development
Software is at the core of all computer systems, and it’s an ever-evolving aspect of computer science. New software solutions are needed practically every day, and that’s where software engineering and development come in.
Software engineers design new programs and work out how to implement them. Developers work on finding novel solutions to practical and theoretical challenges. These two branches of computer science are responsible for helping machines keep up with users’ demands, both privately and professionally.
Human-Computer Interaction and User Experience Design
We might not think much about the way we interact with computers. At least that’s the case if the user experience is done right. Designing the elements that people use in regular interaction reflects how efficiently computer systems work. Without quality user experience or means of interaction, software alone doesn’t serve much purpose.
Networking and Cloud Computing
A standalone computer system is a rarity these days. Networking, the internet, and cloud computing unlocked the full potential of the digital world. Today, computers can do their best when connected online, which is why these aspects of computer science count among the most important today.
Internet of Things and Embedded Systems
The Internet of Things (IoT) refers to a network of interconnected smart devices. This technology makes smart homes possible, but that’s only a small part of what IoT can do. Automated manufacturing, logistics, and numerous other complex systems function on this principle. In a sense, IoT and embedded systems represent the pinnacle of computer science since it brings together all other fields of research.
Tips for a Successful MSc Computer Science Application
Applying for an MSc in Computer Science is a step that shouldn’t be taken lightly. Your application will require careful consideration, particularly regarding the career path you wish to take. It would be best to start with a list of programs that fit your chosen field of research.
Once you have that list, you should narrow the choice according to the specific criteria that we listed here. To recap, those criteria are:
- The institution’s reputation and accreditation
- The curriculum
- Faculty and opportunities for research
- Fees and scholarships/financial aid
- Location and facilities
- Networking opportunities and career support
After you choose the program, it will be time to prepare the strongest application possible. You’ll have the best chances of getting accepted into the program with a well-written statement of purpose, the appropriate letters of recommendation, test scores and academic transcripts, and written proof of extracurricular activities and work experience.
Lastly, you should prepare to visit the campus and schedule an interview. Don’t disregard this aspect of the application process, as it could easily determine whether you’ll get accepted.
Start Your Computer Science Master’s Journey Today
Getting an MSc in Computer Science may be a significant boost for your career. Select the right program, and you might find yourself at the top of the job market. If your interests fall into any field of computer science, consider enrolling in a master’s program at a leading institution – it will be an excellent career move.
Have questions?
Visit our FAQ page or get in touch with us!
Write us at +39 335 576 0263
Get in touch at hello@opit.com
Talk to one of our Study Advisors
We are international
We can speak in: