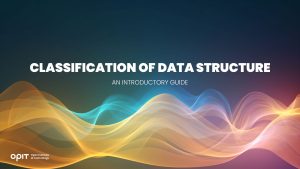
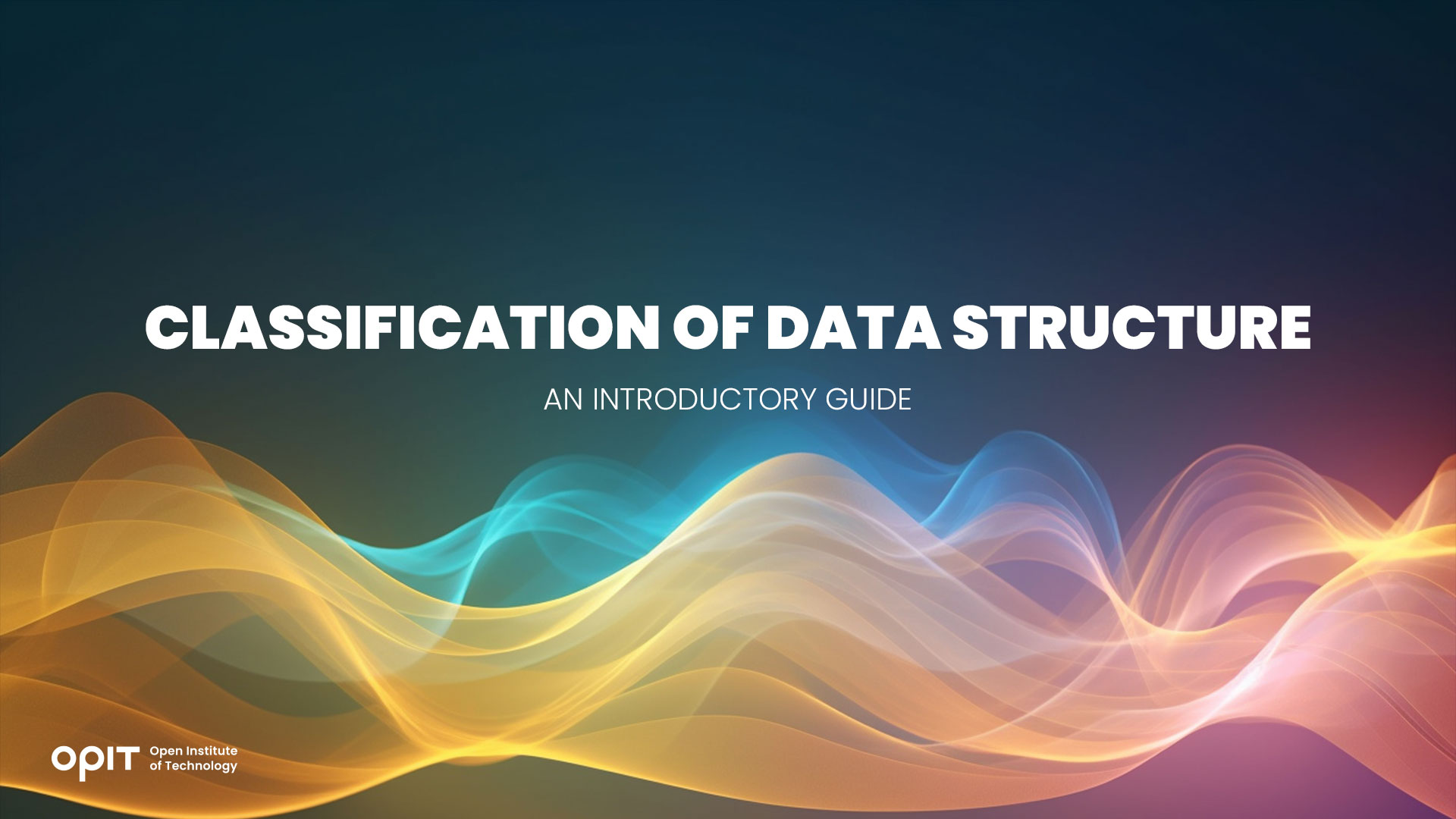
Most people feel much better when they organize their personal spaces. Whether that’s an office, living room, or bedroom, it feels good to have everything arranged. Besides giving you a sense of peace and satisfaction, a neatly-organized space ensures you can find everything you need with ease.
The same goes for programs. They need data structures, i.e., ways of organizing data to ensure optimized processing, storage, and retrieval. Without data structures, it would be impossible to create efficient, functional programs, meaning the entire computer science field wouldn’t have its foundation.
Not all data structures are created equal. You have primitive and non-primitive structures, with the latter being divided into several subgroups. If you want to be a better programmer and write reliable and efficient codes, you need to understand the key differences between these structures.
In this introduction to data structures, we’ll cover their classifications, characteristics, and applications.
Primitive Data Structures
Let’s start our journey with the simplest data structures. Primitive data structures (simple data types) consist of characters that can’t be divided. They aren’t a collection of data and can store only one type of data, hence their name. Since primitive data structures can be operated (manipulated) directly according to machine instructions, they’re invaluable for the transmission of information between the programmer and the compiler.
There are four basic types of primitive data structures:
- Integers
- Floats
- Characters
- Booleans
Integers
Integers store positive and negative whole numbers (along with the number zero). As the name implies, integer data types use integers (no fractions or decimal points) to store precise information. If a value doesn’t belong to the numerical range integer data types support, the server won’t be able to store it.
The main advantages here are space-saving and simplicity. With these data types, you can perform arithmetic operations and store quantities and counts.
Floats
Floats are the opposite of integers. In this case, you have a “floating” number or a number that isn’t whole. They offer more precision but still have a high speed. Systems that have very small or extremely large numbers use floats.
Characters
Next, you have characters. As you may assume, character data types store characters. The characters can be a string of uppercase and/or lowercase single or multibyte letters, numbers, or other symbols that the code set “approves.”
Booleans
Booleans are the third type of data supported by computer programs (the other two are numbers and letters). In this case, the values are positive/negative or true/false. With this data type, you have a binary, either/or division, so you can use it to represent values as valid or invalid.
Linear Data Structures
Let’s move on to non-primitive data structures. The first on our agenda are linear data structures, i.e., those that feature data elements arranged sequentially. Every single element in these structures is connected to the previous and the following element, thus creating a unique linear arrangement.
Linear data structures have no hierarchy; they consist of a single level, meaning the elements can be retrieved in one run.
We can distinguish several types of linear data structures:
- Arrays
- Linked lists
- Stacks
- Queues
Arrays
Arrays are collections of data elements belonging to the same type. The elements are stored at adjoining locations, and each one can be accessed directly, thanks to the unique index number.
Arrays are the most basic data structures. If you want to conquer the data science field, you should learn the ins and outs of these structures.
They have many applications, from solving matrix problems to CPU scheduling, speech processing, online ticket booking systems, etc.
Linked Lists
Linked lists store elements in a list-like structure. However, the nodes aren’t stored at contiguous locations. Here, every node is connected (linked) to the subsequent node on the list with a link (reference).
One of the best real-life applications of linked lists is multiplayer games, where the lists are used to keep track of each player’s turn. You also use linked lists when viewing images and pressing right or left arrows to go to the next/previous image.
Stacks
The basic principles behind stacks are LIFO (last in, first out) or FILO (first in, last out). These data structures stick to a specific order of operations and entering and retrieving information can be done only from one end. Stacks can be implemented through linked lists or arrays and are parts of many algorithms.
With stacks, you can evaluate and convert arithmetic expressions, check parentheses, process function calls, undo/redo your actions in a word processor, and much more.
Queues
In these linear structures, the principle is FIFO (first in, first out). The data the program stores first will be the first to process. You could say queues work on a first-come, first-served basis. Unlike stacks, queues aren’t limited to entering and retrieving information from only one end. Queues can be implemented through arrays, linked lists, or stacks.
There are three types of queues:
- Simple
- Circular
- Priority
You use these data structures for job scheduling, CPU scheduling, multiple file downloading, and transferring data.
Non-Linear Data Structures
Non-linear and linear data structures are two diametrically opposite concepts. With non-linear structures, you don’t have elements arranged sequentially. This means there isn’t a single sequence that connects all elements. In this case, you have elements that can have multiple paths to each other. As you can imagine, implementing non-linear data structures is no walk in the park. But it’s worth it. These structures allow multi-level storage (hierarchy) and offer incredible memory efficiency.
Here are three types of non-linear data structures we’ll cover:
- Trees
- Graphs
- Hash tables
Trees
Naturally, trees have a tree-like structure. You start at the root node, which is divided into other nodes, and end up with leaf modes. Every node has one “parent” but can have multiple “children,” depending on the structure. All nodes contain some type of data.
Tree structures provide easier access to specific data and guarantee efficiency.
Three structures are often used in game development and indexing databases. You’ll also use them in machine learning, particularly decision analysis.
Graphs
The two most important elements of every graph are vertices (nodes) and edges. A graph is essentially a finite collection of vertices connected by edges. Although they may look simple, graphs can handle the most complex tasks. They’re used in operating systems and the World Wide Web.
You unconsciously use graphs with Google Maps. When you want to know the directions to a specific location, you enter it in the map. At that point, the location becomes the node, and the path that guides you is the edge.
Hash Tables
With hash tables, you store information in an associative manner. Every data value gets its unique index value, meaning you can quickly find exactly what you’re looking for.
This may sound complex, so let’s check out a real-life example. Think of a library with over 30,000 books. Every book gets a number, and the librarian uses this number when trying to locate it or learn more details about it.
That’s exactly how hash tables work. They make the search process and insertion much faster, which is why they have a wide array of applications.
Specialized Data Structures
When data structures can’t be classified as either linear or non-linear, they’re called specialized data structures. These structures have unique applications and principles and are used to represent specialized objects.
Here are three examples of these structures:
- Trie
- Bloom Filter
- Spatial Data
Trie
No, this isn’t a typo. “Trie” is derived from “retrieval,” so you can guess its purpose. A trie stores data which you can represent as graphs. It consists of nodes and edges, and every node contains a character that comes after the word formed by the parent node. This means that a key’s value is carried across the entire trie.
Bloom Filter
A bloom filter is a probabilistic data structure. You use it to analyze a set and investigate the presence of a specific element. In this case, “probabilistic” means that the filter can determine the absence but can result in false positives.
Spatial Data Structures
These structures organize data objects by position. As such, they have a key role in geographic systems, robotics, and computer graphics.
Choosing the Right Data Structure
Data structures can have many benefits, but only if you choose the right type for your needs. Here’s what to consider when selecting a data structure:
- Data size and complexity – Some data structures can’t handle large and/or complex data.
- Access patterns and frequency – Different structures have different ways of accessing data.
- Required data structure operations and their efficiency – Do you want to search, insert, sort, or delete data?
- Memory usage and constraints – Data structures have varying memory usages. Plus, every structure has limitations you’ll need to get acquainted with before selecting it.
Jump on the Data Structure Train
Data structures allow you to organize information and help you store and manage it. The mechanisms behind data structures make handling vast amounts of data much easier. Whether you want to visualize a real-world challenge or use structures in game development, image viewing, or computer sciences, they can be useful in various spheres.
As the data industry is evolving rapidly, if you want to stay in the loop with the latest trends, you need to be persistent and invest in your knowledge continuously.
Related posts
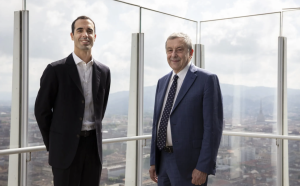
Source:
- EFMD Global, Published on July 12th, 2024.
By Stephanie Mullins
Many people love to read the stories of successful business school graduates to see what they’ve achieved using the lessons, insights and connections from the programmes they’ve studied. We speak to one alumnus, Riccardo Ocleppo, who studied at top business schools including London Business School (LBS) and INSEAD, about the education institution called OPIT which he created after business school.
Please introduce yourself and your career to date.
I am the founder of OPIT — Open Institute of Technology, a fully accredited Higher Education Institution (HEI) under the European Qualification Framework (EQF) by the MFHEA Authority. OPIT also partners with WES (World Education Services), a trusted non-profit providing verified education credential assessments (ECA) in the US and Canada for foreign degrees and certificates.
Prior to founding OPIT, I established Docsity, a global community boasting 15 million registered university students worldwide and partnerships with over 250 Universities and Business Schools. My academic background includes an MSc in Electronics from Politecnico di Torino and an MSc in Management from London Business School.
Why did you decide to create OPIT Open Institute of Technology?
Higher education has a profound impact on people’s futures. Through quality higher education, people can aspire to a better and more fulfilling future.
The mission behind OPIT is to democratise access to high-quality higher education in the fields that will be in high demand in the coming decades: Computer Science, Artificial Intelligence, Data Science, Cybersecurity, and Digital Innovation.
Since launching my first company in the education field, I’ve engaged with countless students, partnered with hundreds of universities, and collaborated with professors and companies. Through these interactions, I’ve observed a gap between traditional university curricula and the skills demanded by today’s job market, particularly in Computer Science and Technology.
I founded OPIT to bridge this gap by modernising education, making it affordable, and enhancing the digital learning experience. By collaborating with international professors and forging solid relationships with global companies, we are creating a dynamic online community and developing high-quality digital learning content. This approach ensures our students benefit from a flexible, cutting-edge, and stress-free learning environment.
Why do you think an education in tech is relevant in today’s business landscape?
As depicted by the World Economic Forum’s “Future of Jobs 2023” report, the demand for skilled tech professionals remains (and will remain) robust across industries, driven by the critical role of advanced technologies in business success.
Today’s companies require individuals who can innovate and execute complex solutions. A degree in fields like computer science, cybersecurity, data science, digital business or AI equips graduates with essential skills to thrive in this dynamic industry.
According to the International Monetary Fund (IMF), the global tech talent shortage will exceed 85 million workers by 2030. The Korn Ferry Institute warns that this gap could result in hundreds of billions in lost revenue across the US, Europe, and Asia.
To address this challenge, OPIT aims to democratise access to technology education. Our competency-based and applied approach, coupled with a flexible online learning experience, empowers students to progress at their own pace, demonstrating their skills as they advance.
Read the full article below:
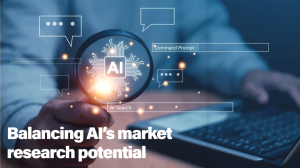
Source:
- The European, Summer 2024 Edition, Page 24
With careful planning, ethical considerations, and ensuring human oversight is maintained, AI can have huge market research benefits, says Lorenzo Livi of the Open Institute of Technology.
By Lorenzo Livi
To market well, you need to get something interesting in front of those who are interested. That takes a lot of thinking, a lot of work, and a whole bunch of research. But what if the bulk of that thinking, work and research could be done for you? What would that mean for marketing as an industry, and market research specifically?
With the recent explosion of AI onto the world stage, big changes are coming in the marketing industry. But will AI be able to do market research as successfully? Simply, the answer is yes. A big, fat, resounding yes. In fact, AI has the potential to revolutionise market research.
Ensuring that people have a clear understanding of what exactly AI is is crucial, given its seismic effect on our world. Common questions that even occur amongst people at the forefront of marketing, such as, “Who invented AI?” or, “Where is the main AI system located?” highlight a widespread misunderstanding about the nature of AI.
As for the notion of a central “main thing” running AI, it’s essential to clarify that AI systems exist in various forms and locations. AI algorithms and models can run on individual computers, servers, or even specialized hardware designed for AI processing, commonly referred to as AI chips. These systems can be distributed across multiple locations, including data centres, cloud platforms, and edge devices. They can also be used anywhere, so long as you have a compatible device and an internet connection.
While the concept of AI may seem abstract or mysterious to some, it’s important to approach it with a clear understanding of its principles and applications. By promoting education and awareness about AI, we can dispel misconceptions and facilitate meaningful conversations about its role in society.
Read the full article below:
- The European, Pages 24 to 26.
Have questions?
Visit our FAQ page or get in touch with us!
Write us at +39 335 576 0263
Get in touch at hello@opit.com
Talk to one of our Study Advisors
We are international
We can speak in: