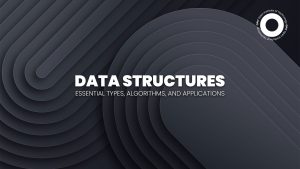
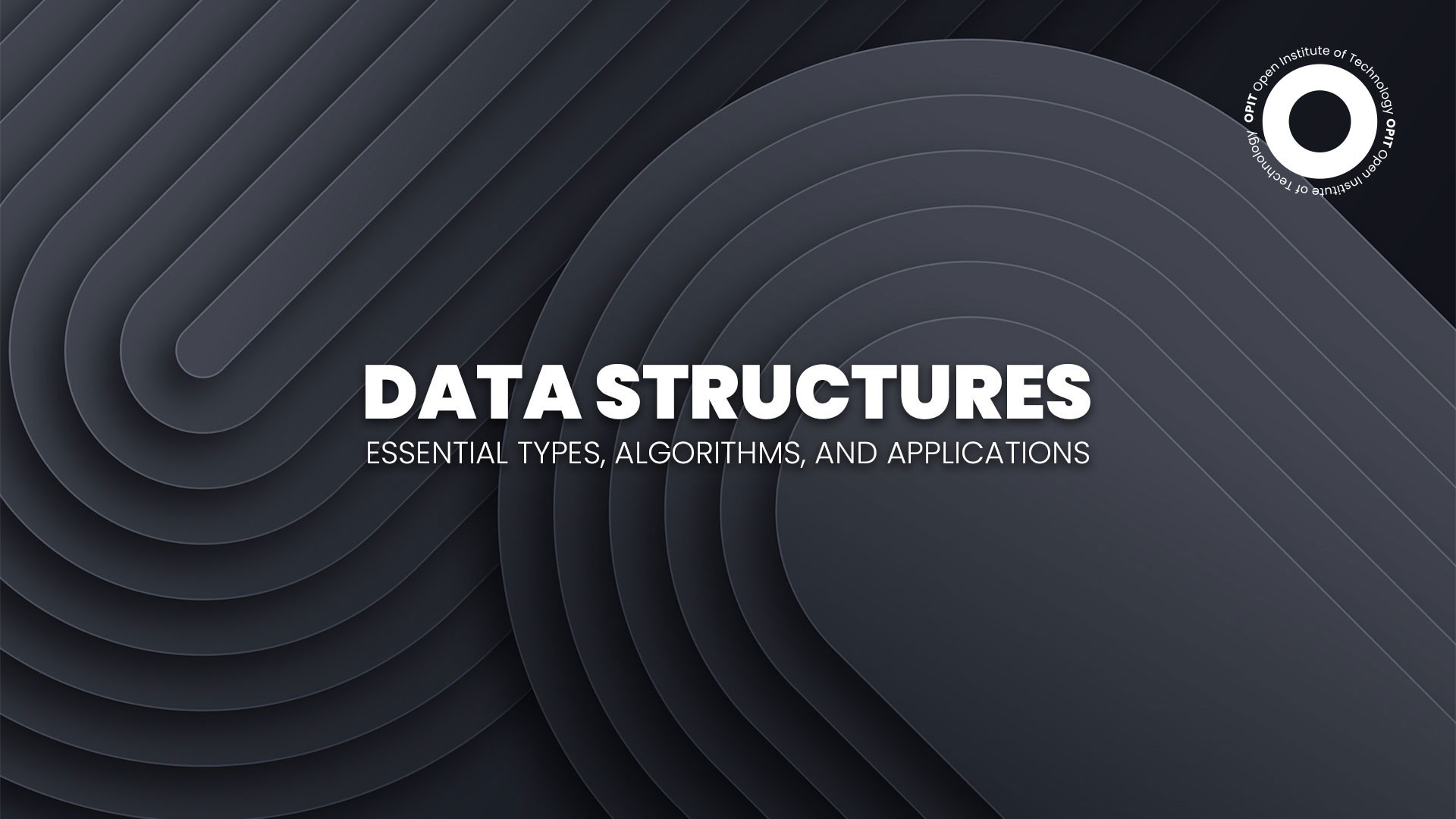
Data is the heartbeat of the digital realm. And when something is so important, you want to ensure you deal with it properly. That’s where data structures come into play.
But what is data structure exactly?
In the simplest terms, a data structure is a way of organizing data on a computing machine so that you can access and update it as quickly and efficiently as possible. For those looking for a more detailed data structure definition, we must add processing, retrieving, and storing data to the purposes of this specialized format.
With this in mind, the importance of data structures becomes quite clear. Neither humans nor machines could access or use digital data without these structures.
But using data structures isn’t enough on its own. You must also use the right data structure for your needs.
This article will guide you through the most common types of data structures, explain the relationship between data structures and algorithms, and showcase some real-world applications of these structures.
Armed with this invaluable knowledge, choosing the right data structure will be a breeze.
Types of Data Structures
Like data, data structures have specific characteristics, features, and applications. These are the factors that primarily dictate which data structure should be used in which scenario. Below are the most common types of data structures and their applications.
Primitive Data Structures
Take one look at the name of this data type, and its structure won’t surprise you. Primitive data structures are to data what cells are to a human body – building blocks. As such, they hold a single value and are typically built into programming languages. Whether you check data structures in C or data structures in Java, these are the types of data structures you’ll find.
- Integer (signed or unsigned) – Representing whole numbers
- Float (floating-point numbers) – Representing real numbers with decimal precision
- Character – Representing integer values as symbols
- Boolean – Storing true or false logical values
Non-Primitive Data Structures
Combine primitive data structures, and you get non-primitive data structures. These structures can be further divided into two types.
Linear Data Structures
As the name implies, a linear data structure arranges the data elements linearly (sequentially). In this structure, each element is attached to its predecessor and successor.
The most commonly used linear data structures (and their real-life applications) include the following:
- In arrays, multiple elements of the same type are stored together in the same location. As a result, they can all be processed relatively quickly. (library management systems, ticket booking systems, mobile phone contacts, etc.)
- Linked lists. With linked lists, elements aren’t stored at adjacent memory locations. Instead, the elements are linked with pointers indicating the next element in the sequence. (music playlists, social media feeds, etc.)
- These data structures follow the Last-In-First-Out (LIFO) sequencing order. As a result, you can only enter or retrieve data from one stack end (browsing history, undo operations in word processors, etc.)
- Queues follow the First-In-First-Out (FIFO) sequencing order (website traffic, printer task scheduling, video queues, etc.)
Non-Linear Data Structures
A non-linear data structure also has a pretty self-explanatory name. The elements aren’t placed linearly. This also means you can’t traverse all of them in a single run.
- Trees are tree-like (no surprise there!) hierarchical data structures. These structures consist of nodes, each filled with specific data (routers in computer networks, database indexing, etc.)
- Combine vertices (or nodes) and edges, and you get a graph. These data structures are used to solve the most challenging programming problems (modeling, computation flow, etc.)
Advanced Data Structures
Venture beyond primitive data structures (building blocks for data structures) and basic non-primitive data structures (building blocks for more sophisticated applications), and you’ll reach advanced data structures.
- Hash tables. These advanced data structures use hash functions to store data associatively (through key-value pairs). Using the associated values, you can quickly access the desired data (dictionaries, browser searching, etc.)
- Heaps are specialized tree-like data structures that satisfy the heap property (every tree element is larger than its descendant.)
- Tries store strings that can be organized in a visual graph and retrieved when necessary (auto-complete function, spell checkers, etc.)
Algorithms for Data Structures
There is a common misconception that data structures and algorithms in Java and other programming languages are one and the same. In reality, algorithms are steps used to structure data and solve other problems. Check out our overview of some basic algorithms for data structures.
Searching Algorithms
Searching algorithms are used to locate specific elements within data structures. Whether you’re searching for specific data structures in C++ or another programming language, you can use two types of algorithms:
- Linear search: starts from one end and checks each sequential element until the desired element is located
- Binary search: looks for the desired element in the middle of a sorted list of items (If the elements aren’t sorted, you must do that before a binary search.)
Sorting Algorithms
Whenever you need to arrange elements in a specific order, you’ll need sorting algorithms.
- Bubble sort: Compares two adjacent elements and swaps them if they’re in the wrong order
- Selection sort: Sorts lists by identifying the smallest element and placing it at the beginning of the unsorted list
- Insertion sort: Inserts the unsorted element in the correct position straight away
- Merge sort: Divides unsorted lists into smaller sections and orders each separately (the so-called divide-and-conquer principle)
- Quick sort: Also relies on the divide-and-conquer principle but employs a pivot element to partition the list (elements smaller than the pivot element go back, while larger ones are kept on the right)
Tree Traversal Algorithms
To traverse a tree means to visit its every node. Since trees aren’t linear data structures, there’s more than one way to traverse them.
- Pre-order traversal: Visits the root node first (the topmost node in a tree), followed by the left and finally the right subtree
- In-order traversal: Starts with the left subtree, moves to the root node, and ends with the right subtree
- Post-order traversal: Visits the nodes in the following order: left subtree, right subtree, the root node
Graph Traversal Algorithms
Graph traversal algorithms traverse all the vertices (or nodes) and edges in a graph. You can choose between two:
- Depth-first search – Focuses on visiting all the vertices or nodes of a graph data structure located one above the other
- Breadth-first search – Traverses the adjacent nodes of a graph before moving outwards
Applications of Data Structures
Data structures are critical for managing data. So, no wonder their extensive list of applications keeps growing virtually every day. Check out some of the most popular applications data structures have nowadays.
Data Organization and Storage
With this application, data structures return to their roots: they’re used to arrange and store data most efficiently.
Database Management Systems
Database management systems are software programs used to define, store, manipulate, and protect data in a single location. These systems have several components, each relying on data structures to handle records to some extent.
Let’s take a library management system as an example. Data structures are used every step of the way, from indexing books (based on the author’s name, the book’s title, genre, etc.) to storing e-books.
File Systems
File systems use specific data structures to represent information, allocate it to the memory, and manage it afterward.
Data Retrieval and Processing
With data structures, data isn’t stored and then forgotten. It can also be retrieved and processed as necessary.
Search Engines
Search engines (Google, Bing, Yahoo, etc.) are arguably the most widely used applications of data structures. Thanks to structures like tries and hash tables, search engines can successfully index web pages and retrieve the information internet users seek.
Data Compression
Data compression aims to accurately represent data using the smallest storage amount possible. But without data structures, there wouldn’t be data compression algorithms.
Data Encryption
Data encryption is crucial for preserving data confidentiality. And do you know what’s crucial for supporting cryptography algorithms? That’s right, data structures. Once the data is encrypted, data structures like hash tables also aid with value key storage.
Problem Solving and Optimization
At their core, data structures are designed for optimizing data and solving specific problems (both simple and complex). Throw their composition into the mix, and you’ll understand why these structures have been embraced by fields that heavily rely on mathematics and algorithms for problem-solving.
Artificial Intelligence
Artificial intelligence (AI) is all about data. For machines to be able to use this data, it must be properly stored and organized. Enter data structures.
Arrays, linked lists, queues, graphs, and stacks are just some structures used to store data for AI purposes.
Machine Learning
Data structures used for machine learning (MI) are pretty similar to other computer science fields, including AI. In machine learning, data structures (both linear and non-linear) are used to solve complex mathematical problems, manipulate data, and implement ML models.
Network Routing
Network routing refers to establishing paths through one or more internet networks. Various routing algorithms are used for this purpose and most heavily rely on data structures to find the best patch for the incoming data packet.
Data Structures: The Backbone of Efficiency
Data structures are critical in our data-driven world. They allow straightforward data representation, access, and manipulation, even in giant databases. For this reason, learning about data structures and algorithms further can open up a world of possibilities for a career in data science and related fields.
Related posts
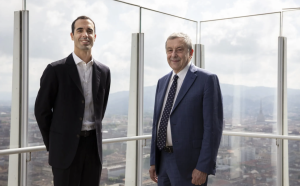
Source:
- EFMD Global, Published on July 12th, 2024.
By Stephanie Mullins
Many people love to read the stories of successful business school graduates to see what they’ve achieved using the lessons, insights and connections from the programmes they’ve studied. We speak to one alumnus, Riccardo Ocleppo, who studied at top business schools including London Business School (LBS) and INSEAD, about the education institution called OPIT which he created after business school.
Please introduce yourself and your career to date.
I am the founder of OPIT — Open Institute of Technology, a fully accredited Higher Education Institution (HEI) under the European Qualification Framework (EQF) by the MFHEA Authority. OPIT also partners with WES (World Education Services), a trusted non-profit providing verified education credential assessments (ECA) in the US and Canada for foreign degrees and certificates.
Prior to founding OPIT, I established Docsity, a global community boasting 15 million registered university students worldwide and partnerships with over 250 Universities and Business Schools. My academic background includes an MSc in Electronics from Politecnico di Torino and an MSc in Management from London Business School.
Why did you decide to create OPIT Open Institute of Technology?
Higher education has a profound impact on people’s futures. Through quality higher education, people can aspire to a better and more fulfilling future.
The mission behind OPIT is to democratise access to high-quality higher education in the fields that will be in high demand in the coming decades: Computer Science, Artificial Intelligence, Data Science, Cybersecurity, and Digital Innovation.
Since launching my first company in the education field, I’ve engaged with countless students, partnered with hundreds of universities, and collaborated with professors and companies. Through these interactions, I’ve observed a gap between traditional university curricula and the skills demanded by today’s job market, particularly in Computer Science and Technology.
I founded OPIT to bridge this gap by modernising education, making it affordable, and enhancing the digital learning experience. By collaborating with international professors and forging solid relationships with global companies, we are creating a dynamic online community and developing high-quality digital learning content. This approach ensures our students benefit from a flexible, cutting-edge, and stress-free learning environment.
Why do you think an education in tech is relevant in today’s business landscape?
As depicted by the World Economic Forum’s “Future of Jobs 2023” report, the demand for skilled tech professionals remains (and will remain) robust across industries, driven by the critical role of advanced technologies in business success.
Today’s companies require individuals who can innovate and execute complex solutions. A degree in fields like computer science, cybersecurity, data science, digital business or AI equips graduates with essential skills to thrive in this dynamic industry.
According to the International Monetary Fund (IMF), the global tech talent shortage will exceed 85 million workers by 2030. The Korn Ferry Institute warns that this gap could result in hundreds of billions in lost revenue across the US, Europe, and Asia.
To address this challenge, OPIT aims to democratise access to technology education. Our competency-based and applied approach, coupled with a flexible online learning experience, empowers students to progress at their own pace, demonstrating their skills as they advance.
Read the full article below:
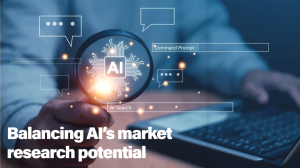
Source:
- The European, Summer 2024 Edition, Page 24
With careful planning, ethical considerations, and ensuring human oversight is maintained, AI can have huge market research benefits, says Lorenzo Livi of the Open Institute of Technology.
By Lorenzo Livi
To market well, you need to get something interesting in front of those who are interested. That takes a lot of thinking, a lot of work, and a whole bunch of research. But what if the bulk of that thinking, work and research could be done for you? What would that mean for marketing as an industry, and market research specifically?
With the recent explosion of AI onto the world stage, big changes are coming in the marketing industry. But will AI be able to do market research as successfully? Simply, the answer is yes. A big, fat, resounding yes. In fact, AI has the potential to revolutionise market research.
Ensuring that people have a clear understanding of what exactly AI is is crucial, given its seismic effect on our world. Common questions that even occur amongst people at the forefront of marketing, such as, “Who invented AI?” or, “Where is the main AI system located?” highlight a widespread misunderstanding about the nature of AI.
As for the notion of a central “main thing” running AI, it’s essential to clarify that AI systems exist in various forms and locations. AI algorithms and models can run on individual computers, servers, or even specialized hardware designed for AI processing, commonly referred to as AI chips. These systems can be distributed across multiple locations, including data centres, cloud platforms, and edge devices. They can also be used anywhere, so long as you have a compatible device and an internet connection.
While the concept of AI may seem abstract or mysterious to some, it’s important to approach it with a clear understanding of its principles and applications. By promoting education and awareness about AI, we can dispel misconceptions and facilitate meaningful conversations about its role in society.
Read the full article below:
- The European, Pages 24 to 26.
Have questions?
Visit our FAQ page or get in touch with us!
Write us at +39 335 576 0263
Get in touch at hello@opit.com
Talk to one of our Study Advisors
We are international
We can speak in: